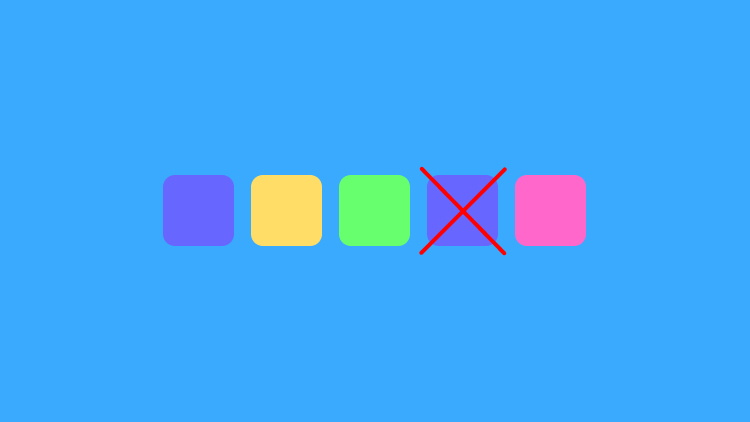
Introduction to SF Symbols
swift swiftui xcode images icons sf symbols
SF Symbols is a library of over 6,000 symbols that are designed to integrate seamlessly with San Francisco, the system font for Apple platforms.
Introduction
As noted, SF Symbols are vector-based icons that Apple provides us access to that are scalable. These images adapt to various weights, sizes and can even be animated to add personality and a positive user experience.
import SwiftUI
struct ContentView: View {
var body: some View {
Image(systemName: "bell")
}
}
When using SF Symbols, it is highly recommended to refer to the Apple Human Interface Guidelines to see the best use cases to ensure the best look and feel of the application while remaining accessible.
Sizing
When using SF Symbols, we’ll display them using an Image(systemName: String)
object however we will treat it more like text when it comes to customization. With that being said, to adjust the size of the icon we’ll use the .font()
modifier applied to the image.
VStack {
Image(systemName: "bell")
.font(.title)
Image(systemName: "bell")
.font(.headline)
Image(systemName: "bell")
.font(.caption)
}
Since we can modify the symbol much like we can with text, that means we can change the weight of the symbol as well to add some emphasis on the icon itself or what it’s attempting to symbolize.
VStack {
Image(systemName: "bell")
.fontWeight(.light)
Image(systemName: "bell")
.fontWeight(.bold)
Image(systemName: "bell")
.fontWeight(.black)
}
When we need to scale the icon relative to the font size, we can use the .imageScale
modifier. There are three options that can be used which are .small
, .medium
, and .large
, which scale the icon proportionally to the font size. If the font is not set explicitly, the symbol will inherit the font from the current environment.
Note: It’s not recommended to use the .resizable()
modifier.
When .reiszable()
is used, the image stops being a symbol image and can negatively impact it’s ability to conform to the layout and alignment with text.
Color
Customizing the color of an SF Symbol is fairly straight forward as we can use the .foregroundStyle
modifier. The benefit to being able to use .foregroundStyle
is it allows us to apply any ShapeStyle
, including gradients, which opens up a wide range of customization possibilities for our symbol images.
Image(systemName: "bell")
.foregroundStyle(.red)
Image(systemName: "bell")
.foregroundStyle(
LinearGradient(
colors: [.yellow, .red],
startPoint: .top,
endPoint: .bottom
)
)
Hopefully this brief introduction has been helpful in aiding in your development. If you have any specific topics you’d like covered, feel free to reach out on X or via email.