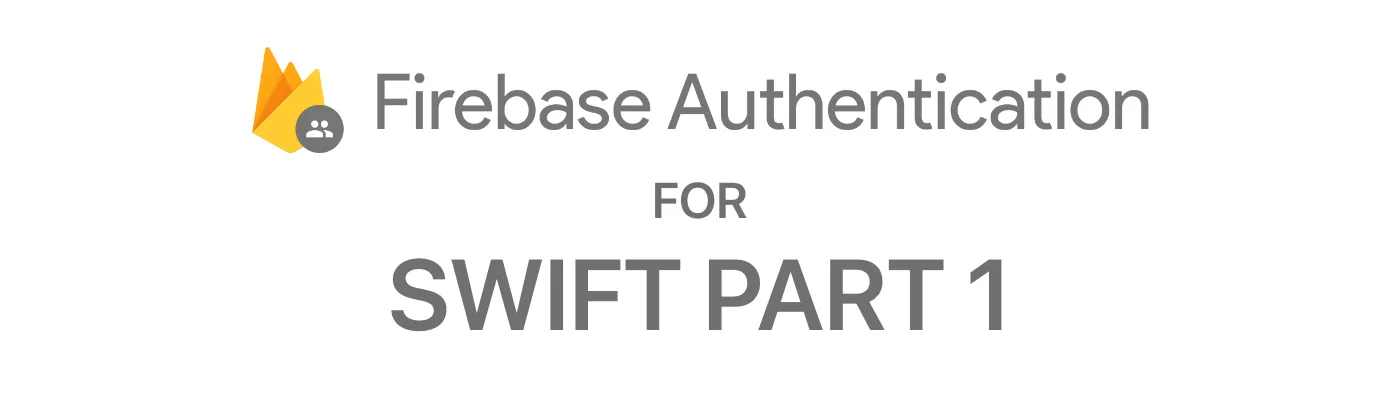
Firebase Authentication for Swift - Part 1
What is Firebase?
It depends on who you ask… For some, it is a fantastic platform that allows for easy integration of authentication, real-time database, or even cloud database-style storage. Others side with Mama Bouchard from The Waterboy and think it’s the devil.
Firebase is Google’s mobile application development platform that helps you build, improve, and grow your app. — Google
As an indie developer with a little over a year of experience, elbow-deep in a very large project, Firebase has been a wonderful, easy-to-use platform that has allowed me to use their authentication and Firestore products. With that being said, I’ve had my fair share of hurdles, hiccups, and rookie mistakes. This series aims to document some of those for others as well as myself.
To learn a more in-depth view of Firebase and its use cases, I highly recommend you check out the Firebase website. As you poke around, you should find a good bit of information.
Getting Started with Firebase Authentication
Once you get yourself signed up and signed in, you’ll head to your console and add a project. Onboarding is fairly simple, you’ll follow the instructions and continue on until you’re at your projects console — I won’t bore you to death with that stuff as I have faith that you’re smart enough to figure out those portions.
Once you’ve done that, we’ll jump into Xcode and get to implementing Firebase Authentication into your project.
Importing and Initial Xcode Project Setup
Once you’ve got Xcode open and created your project, it becomes time to import Firebase and its friends into your project. This is a blessing and a curse… It’s fairly easy, but you’re about to quickly become a real developer as build times are going to become longer, Xcode is going to begin throwing a fit and the fun will begin.
As you will see above, we need to navigate to our projects Package Dependencies window and click the + to add a package. From there, we will focus on using Swift Package Manager (SPM) to add Firebase to our project.
To do this, travel to the Firebase Github to get information from Google however if you run into an issue feel free to reach out to me on Twitter and I’ll lend you a hand.
You’ll be prompted with a long list of checkboxes to select what you’d like to add, for our project you’ll add FirebaseAuth
, FirebaseFirestore
, and FirebaseFirestore-Swift
. Once you’ve added Firebase to your project, go get a coffee, some water… it’s going to import and build. This takes time… unfortunately, building usually takes more time than we like. Once the stars align, we’re ready to fire up Authentication on the Firebase console, I’ll meet you there.
Firebase Console — Authentication & Firestore Initial Setup
On your console, you’ll see on the left Firebase Authentication select that and click Get Started, once that is complete select Email / Password and enable it as that is the method we will focus on.
We’re also going to add the Firebase Firestore, this will allow us to save more information such as name, address, or whatever else besides email and password. Once you’ve selected Firebase Firestore, follow the prompts — you’ve got this.
IMPORTANT — BE SURE TO CHECK COPY ITEMS IF NEEDED.
The reason we want to check copy items if needed is because when we click and drag the Google-Info.plist
to add to our project, Xcode creates a reference to that file. If that file gets deleted and there isn’t a copy created — Xcode is going to go have a fit worse then a toddler during terrible twos not getting their way.
Let’s initialize, shall we… Navigate to our applications main app file, this is typically the name of our application — in my case it is called MediumArticleApp
. Once there, we’re going to add an initializer for Firebase so every time our project launches, Firebase fires up ready to work.
import SwiftUI
import Firebase
@main
struct MediumArticleApp: App {
init() {
FirebaseApp.configure()
}
var body: some Scene {
WindowGroup {
ContentView()
}
}
}
Wrapping Up
Just like that we created a project on Firebase, we’ve integrated it into our Xcode project using Swift Package Manager (SPM) and we’ve initialized Firebase in our project. In Part 2, we’ll look into writing up our authentication service so we can begin registering users.